This is a barebone CMake setup for building a C++ project that uses RayLib.
NOTE: Be sure that the RAYLIB_DIR variable in the CMake points to where your RayLib root directory is (this will be where RayLib’s CMakeLists.txt exists).
# Minimum version of cmake required
cmake_minimum_required(VERSION 3.25)
# C++ Version
set(CMAKE_CXX_STANDARD 20)
# Set project name
project(
project_name
VERSION 1.0.0
DESCRIPTION "Project Name"
)
# Set the projects root directory
message ("\n****************************************")
set(ROOT_DIR ${PROJECT_SOURCE_DIR})
message (" Project Name: ${PROJECT_NAME} \n Root Directory: ${ROOT_DIR}")
message ("****************************************")
# Thirdparty directory
set(THIRDPARTY_DIR thirdparty)
set(RAYLIB_DIR ${THIRDPARTY_DIR}/raylib/raylib)
message ("\n****************************************")
message (" RayLib Directory: ${RAYLIB_DIR}")
message ("****************************************")
add_subdirectory(${RAYLIB_DIR})
# Set sources for project
set(projectSOURCES
main.cpp
# insert .cpp's here
)
# Set headers for project
set(projectHEADERS
# insert .hpp's here
)
# Create executable target
add_executable(${PROJECT_NAME}
${projectSOURCES}
${projectHEADERS}
)
# Link libraries
target_link_libraries(${PROJECT_NAME}
raylib
)
# Set the install directory for the executable
install(TARGETS ${PROJECT_NAME}
CONFIGURATIONS Debug
RUNTIME DESTINATION Debug/bin
)
install(TARGETS ${PROJECT_NAME}
CONFIGURATIONS Release
RUNTIME DESTINATION Release/bin
)
A small main.cpp to go along with the CMake above that will create a window using RayLib.
#include <iostream>
#include "raylib.h"
// ----------------------------------------------------------------------------
// main
// ----------------------------------------------------------------------------
int main() {
std::cout << "Main" << std::endl;
// Variables
const int FPS_RATE{ 60 };
// Window Parameters
const int windowWidth{ 800 };
const int windowHeight{ 450 };
InitWindow(windowWidth, windowHeight, "Endless Runner");
Color backgroundColor{ WHITE };
SetTargetFPS(FPS_RATE);
// Delta time
float dt{};
// Game loop
while (!WindowShouldClose()) {
dt = GetFrameTime();
// Handle input
// user controls
// Handle game state
// game physics
// Handle output
// rendering
BeginDrawing();
ClearBackground(WHITE);
EndDrawing();
}
CloseWindow();
return 0;
}
Example of my RayLib Directory Structure
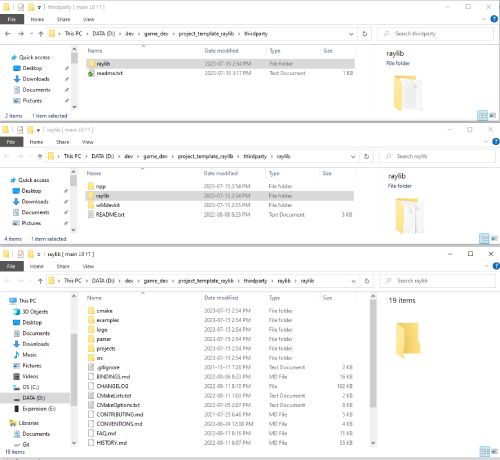
More Complex Version of a RayLib Project
I made a basic C++ project template using RayLib that is a bit more complex than the version above, but, it will set you up for larger projects.
Root Directory CMake
# Minimum version of cmake required
cmake_minimum_required(VERSION 3.25)
# C++ Version
set(CMAKE_CXX_STANDARD 20)
# Set project name
project(
project_name
VERSION 1.0.0
DESCRIPTION "Project Name"
)
# Set the projects root directory
message ("\n****************************************")
set(ROOT_DIR ${PROJECT_SOURCE_DIR})
message (" Project Name: ${PROJECT_NAME} \n Root Directory: ${ROOT_DIR}")
message ("****************************************")
# Thirdparty directory
set(THIRDPARTY_DIR thirdparty)
set(RAYLIB_DIR ${THIRDPARTY_DIR}/raylib/raylib)
message ("\n****************************************")
message (" RayLib Directory: ${RAYLIB_DIR}")
message ("****************************************")
add_subdirectory(${RAYLIB_DIR})
# Add subdirectories
add_subdirectory(code)
Code Directory CMake
# Set project name
project(
main_game
VERSION 1.0.0
DESCRIPTION "Main Game"
)
# Set the projects root directory
message ("\n****************************************")
set(CODE_DIR ${PROJECT_SOURCE_DIR})
message (" Project Name: ${PROJECT_NAME} \n Root Directory: ${CODE_DIR}")
message ("****************************************")
# Set sources for project
set(projectSOURCES
main_game.cpp
MainGame.cpp
)
# Set headers for project
set(projectHEADERS
MainGame.hpp
)
# Create executable target
add_executable(${PROJECT_NAME}
${projectSOURCES}
${projectHEADERS}
)
# Link libraries
target_link_libraries(${PROJECT_NAME}
raylib
)
# Set the install directory for the executable
install(TARGETS ${PROJECT_NAME}
CONFIGURATIONS Debug
RUNTIME DESTINATION Debug/bin
)
install(TARGETS ${PROJECT_NAME}
CONFIGURATIONS Release
RUNTIME DESTINATION Release/bin
)